Centrify SAML Toolkits for SSO
SAML is an XML-based standard for web browser single sign-on (SSO). SAML enables end users to log into websites using authentication from a single Identity Provider (IdP) such as Google, Facebook, and Twitter, thereby eliminating site- and application-specific passwords.
SAML uses single-use, expiring, digital "tokens" to exchange authentication and authorization data between an identity provider and cloud application service provider that have an established trust relationship.
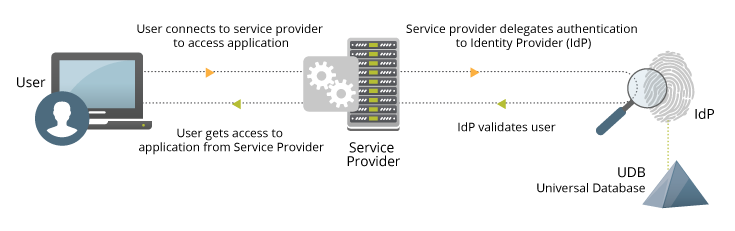
You can use the following toolkits to integrate SAML SSO capabilities into your application or website.
CentrifySAMLSDK_CS
The CentrifySAMLSDK_CS toolkit provides an example that shows you how to integrate SAML with your ASP.NET/C# web application in Visual Studio.
Getting Started with the CentrifySAMLSDK_CS Toolkit
Use the following steps to get started with the toolkit:
- Ensure that Visual Studio is installed and open.
- Clone or download the CentrifySAMLSDK_CS toolkit.
- Open ./SAMLSDK_CS.sln from the toolkit in Visual Studio,
- Navigate to the Centrify Cloud Manager and click on Apps > New App > Custom > SAML Application.
- Click Trust and select Manual Configuration in the Identification Provider Configuration section.
- Expand the Signing Certificate field and click Download.
- Rename the downloaded certificate to SignCertFromCentrify.cer move it to the ./static/certificates/ directory of the toolkit obtained in Step 2, replacing the exiting file in that location.
- Return to the Centrify Cloud Manager, expand the IdP Entity ID / Issuer field, and click Copy.
- Open Web.config in Visual Studio.
- Replace the URL in the following code in Web.config with the URL copied in Step 8:
<configuration>
...
<appSettings>
...
<add key="Issuer" value="https://cloud.centrify.com/SAML/GenericSAML"/>
...
</appSettings>
</configuration>
- Return to the Centrify Cloud Manager, locate the Single Sign On URL field, and click Copy.
- Replace the URL in following line of code in Web.config with the URL copied in Step 11:
<configuration>
...
<appSettings>
...
<add key="IdentityProviderSigninURL" value="https://pod.centrify.com/applogin/appKey/2dd2a829-9145-4100-8c71-16ada9e2e016/customerId/AAA3021"/>
...
</appSettings>
</configuration>
- Return to the Centrify Cloud Manager, navigate to the Server Provider Configuration section on the Trust screen, select Manual Configuration, and set the Assertion Consumer Service (ACS) URL to your localhost and the ACS.aspx page (e.g.
http://localhost:7180/ACS.aspx
). - Deploy the SAML web application in the Centrify Cloud Manager by navigating to the Permissions screen, assigning a user, group or role, and then saving the app.
- Click Debug in Visual Studio. This will open a browser and redirect it to the login screen. If you navigate to Default.aspx, this will start SP Initiated SAML SSO. If you go the User Portal and click the Generic SAML Application you will start IDP Initiated SAML SSO.
Using the C# SDK
The Toolkit consists of two classes defined in ./SAMLSDK_CS/SAML_Interface.cs that demonstrate how to create and decode SAML requests and responses:
SAMLRequest
: defines a method calledGetSAMLRequest
that takes in the ACS URL and issuer, and returns the corresponding SAML request:
public class SAMLRequest
{
public string GetSAMLRequest(string strACSUrl, string strIssuer)
...
}
SAML_Response
: defines three methods to handle SAML responses:ParseSAMLResponse
: takes in an encoded SAML response, decodes it, and returns the raw response in an XML DOM object:
public XmlDocument ParseSAMLResponse(string strEncodedSAMLResponse)
{
...
}
IsResponseValid
: takes in an XML version of the response and indicates if the response has been properly signed based on the certificate:
public bool IsResponseValid(XmlDocument xDoc)
{
...
}
ParseSAMLNameID
: extracts the ID of the authenticated user from the XML response:
public string ParseSAMLNameID(XmlDocument xDoc)
{
...
}
The usage of the SAMLRequest
class can be seen in the Default
class defined in ./SAMLSDK_CS/Default.aspx.cs. When the page_load
event is invoked, it constructs the SAML request using the ACS URL and redirects the user to the sign in page:
public partial class Default : System.Web.UI.Page
{
...
protected void Page_Load(object sender, EventArgs e)
{
SAMLRequest request = new SAMLRequest();
Response.Redirect(IdentityProviderSigninURL + "?SAMLRequest=" + Server.UrlEncode(request.GetSAMLRequest(ACSURL, Issuer)));
}
}
The ACS page, defined by the Default
class in ./SAMLSDK_CS/ACS.aspx.cs, gets a SAML response that it passes to ParseSAMLResponse
. The output from ParseSAMLResponse
is converted to an XML DOM object, and that DOM is passed to IsResponseValid
to check that it contains a valid SAML response. If the response is valid, then ParseSAMLNameID
is invoked to extract the authenticated user ID and include it in a string message:
public partial class Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
SAMLResponse samlResponse = new SAMLResponse();
XmlDocument xDoc = samlResponse.ParseSAMLResponse(Request.Form["SAMLResponse"]);
if (samlResponse.IsResponseValid(xDoc))
{
Response.Write("SAML Response from IDP Was Accepted. Authenticated user is " + samlResponse.ParseSAMLNameID(xDoc));
}
else
{
Response.Write("SAML Response from IDP Was Not Accepted");
}
}
}
CentrifySAMLSDK_Rails
The CentrifySAMLSDK_Rails toolkit provides an example that shows you how to add SAML to your Ruby on Rails web application.
Getting Started with the CentrifySAMLSDK_Rails Toolkit
Use the following steps to get started with the toolkit:
- Ensure that Ruby and Rails are installed.
- Clone or download the CentrifySAMLSDK_Rails toolkit.
- Navigate to the Centrify Cloud Manager and click on Apps > New App > Custom > SAML Application.
- Click Trust and select Manual Configuration in the Identification Provider Configuration section.
- Expand the Signing Certificate field and click Download.
- Move the downloaded .cer file to the root of the toolkit obtained in Step 2.
- Return to the Centrify Cloud Manager, expand the IdP Entity ID / Issuer field, and click Copy.
- Open ./app/controllers/saml_controller.rb in a text editor.
- Replace the second URL in the following code with the URL copied in Step 7:
base64request = newRequest.GetSAMLRequest("http://localhost:3000/saml/acs", "https://cloud.centrify.com/SAML/GenericSAML")
- Return to the Centrify Cloud Manager, locate the Single Sign On URL field, and click Copy.
- Replace the URL in following line of code from ./app/controllers/saml_controller.rb with the URL copied in Step 10:
redirect_to generate_url("https://aaa3021.my-kibble.centrify.com/applogin/appKey/ac53efff-d0e4-4532-9faf-547bafc1a1f7/customerId/AAA3021", :SAMLRequest => base64request)
- Modify the following line replacing the .cer filename with the name of the .cer file you downloaded:
certificate = OpenSSL::X509::Certificate.new(File.read("SignCertFromCentrify.cer"))
- Return to the Centrify Cloud Manager, navigate to the Server Provider Configuration section on the Trust screen, select Manual Configuration, and set the Assertion Consumer Service (ACS) URL to your localhost and the ACS.aspx page (e.g.
http://localhost:3000/saml/acs
). - Deploy the SAML web application in the Centrify Cloud Manager by navigating to the Permissions screen, assigning a user, group or role, and then saving the app.
- Open a terminal window, navigate to the bin folder within the toolkit and start the server with the following command:
rails server
- Open a browser and navigate to
http://localhost:3000
. This starts SP initiated SAML SSO. If you navigate to the user portal and click the SAML Application this will start IDP initiated SAML SSO.
Using the Ruby SDK
The Toolkit consists of two classes defined in ./app/controllers/saml_controller.rb that demonstrate how to create and decode SAML requests and responses:
SAML_Request
: defines a method calledGetSAMLRequest
that takes in the ACS URL and issuer, and returns the corresponding SAML request:
class SAMLRequest
def GetSAMLRequest(strACSUrl, strIssuer)
...
SAML_Response
: defines three methods to handle SAML responses:ParseSAMLResponse
: takes in an encoded SAML response, decodes it, and returns the raw response:
def ParseSAMLResponse(saml)
...
IsResponseValid
: takes in an XML version of the response and indicates if the response has been properly signed:
def IsResponseValid?(xDoc)
...
ParseNameId
: extracts the ID of the authenticated user from the XML response:
def ParseNameId(xDoc)
...
The usage of these methods can be seen in the SamlController
class defined in ./app/controllers/saml_controller.rb. The default route constructs the various URLs, invokes GetSAMLRequest
, and uses the response to redirect the user:
def default
newRequest = SAMLRequest.new
base64request = newRequest.GetSAMLRequest("http://localhost:3000/saml/acs", "https://aaa3226.my-kibble.centrify.com/4a343909-0397-450a-91f8-5bb932da5711")
redirect_to generate_url("https://aaa3226.my-kibble.centrify.com/applogin/appKey/4a343909-0397-450a-91f8-5bb932da5711/customerId/AAA3226", :SAMLRequest => base64request)
end
The ACS route receives a SAML response that it passes to ParseSAMLResponse
. The output from ParseSAMLResponse
is converted to an XML DOM using Nokogiri, and that DOM is passed to IsResponseValid
to check that it has been properly signed using the certificate. If the response is valid, then ParseNameId
is invoked to extract the authenticated user ID and include it in a string message:
def acs
samlResponse = SAMLResponse.new
strCleanResponse = samlResponse.ParseSAMLResponse(params[:SAMLResponse])
xDoc = Nokogiri::XML(strCleanResponse)
if samlResponse.IsResponseValid?(xDoc)
@greeting = "SAML Response from IDP Was Accepted. Authenticated user is " + samlResponse.ParseNameId(xDoc)
else
@greeting = "SAML Response from IDP Was Not Accepted"
end
end
CentrifySAMLSDK_PyBottle
The CentrifySAMLSDK_PyBottle toolkit provides an example that shows how to add SAML to your Python web application. This example uses Bottle, but the code will work with any framework.
Getting Started with the CentrifySAMLSDK_PyBottle Toolkit
Use the following steps to get started with the toolkit:
- Ensure that Python is installed.
- Ensure that
lxml
,signxml
, andbottle
are installed:
pip install lxml
pip install signxml
pip install bottle
- Clone or download the CentrifySAMLSDK_PyBottle toolkit.
- Navigate to the Centrify Cloud Manager and click on Apps > New App > Custom > SAML Application.
- Click Trust and select Manual Configuration in the Identification Provider Configuration section.
- Expand the Signing Certificate field and click Download.
- Rename the downloaded .cer file to
SignCertFromCentrify.cer
and replace the existing .cer file in the /static/certificates directory with the downloaded/renamed .cer file. - Return to the Centrify Cloud Manager, expand the IdP Entity ID / Issuer field, and click Copy.
- Open ./SAMLSDK_PyBottle.py in a text editor.
- Replace the URL in the following line of code with the URL copied in Step 8:
strIssuer = 'https://cloud.centrify.com/SAML/GenericSAML'
- Return to the Centrify Cloud Manager, locate the Single Sign On URL field, and click Copy.
- Replace the URL in following line of code from ./SAMLSDK_PyBottle.py with the URL copied in Step 11:
strIdentityProviderSigninURL = 'https://aaa3021.my-kibble.centrify.com/applogin/appKey/583e4d88-a193-4ea8-b306-e053b7fe2b7b/customerId/AAA3021'
- Return to the Centrify Cloud Manager, navigate to the Server Provider Configuration section on the Trust screen, select Manual Configuration, and set the Assertion Consumer Service (ACS) URL to your localhost and the ACS.aspx page (e.g.
http://localhost:6321/acs
).Also set the SP Entity ID to the same value. - Deploy the SAML web application in the Centrify Cloud Manager by navigating to the Permissions screen, assigning a user, group or role, and then saving the app.
- Open a terminal window, navigate to the root directory within the toolkit, and start the server with the following command:
python SAMLSDK_PyBottle.py
- Open a browser and navigate to
http://localhost:6321/
. This starts SP initiated SAML SSO. If you navigate to the user portal and click the SAML Application this will start IDP initiated SAML SSO.
Using the PyBottle SDK
The Toolkit consists of two classes defined in ./SAML_Interface.py that demonstrate how to create and decode SAML requests and responses:
SAML_Request
: defines a method calledGetSAMLRequest
that takes in the ACS URL and issuer, and returns the corresponding SAML request:
class SAML_Request:
def GetSAMLRequest(strACSUrl = None, strIssuer = None)
...
SAML_Response
: defines a method calledParseSAMLResponse
that takes in an ACS URL and an encoded SAML response, and returns a message indicating who the authenticated user is:
class SAML_Response:
def ParseSAMLResponse(strACSUrl, strEncodedSAMLResponse):
...
The usage of these methods can be seen in ./SAMLSDK.PyBottle.py. The default
route constructs the various URLs, invokes GetSAMLRequest
, and passes the response to bottle to redirect the user:
#Defult
@route('/')
@route('/default')
@view('default')
def default():
...
bottle.redirect('{uIDPUrl}?{bParams}'.format(uIDPUrl = strIdentityProviderSigninURL, bParams = SAML_Interface.SAML_Request.GetSAMLRequest(strAcsUrl, strIssuer)))
The ACS
route receives a SAML response that it passes along with the ACS URL to ParseSAMLResponse
. The value returned from the method is then stored in a message for display:
@route('/acs', method=['GET','POST'])
@view('acs')
def acs():
samlResponse = request.forms['SAMLResponse']
strAcsUrl = 'http://localhost:6321/acs'
return dict(
title='ACS',
message=SAML_Interface.SAML_Response.ParseSAMLResponse(strAcsUrl,samlResponse),
year=datetime.now().year
)
SAML SDK
The [SAML SDK] (https://github.com/centrify/saml-sdk) toolkit provides an example that shows you how to integrate SAML with your Java/J2EE apps.
SimpleSAMLphp
The SimpleSAMLphp module enables you to integrate SAML with your PHP apps.
Updated over 4 years ago